A Guide to Page Transition Animations in SvelteKit
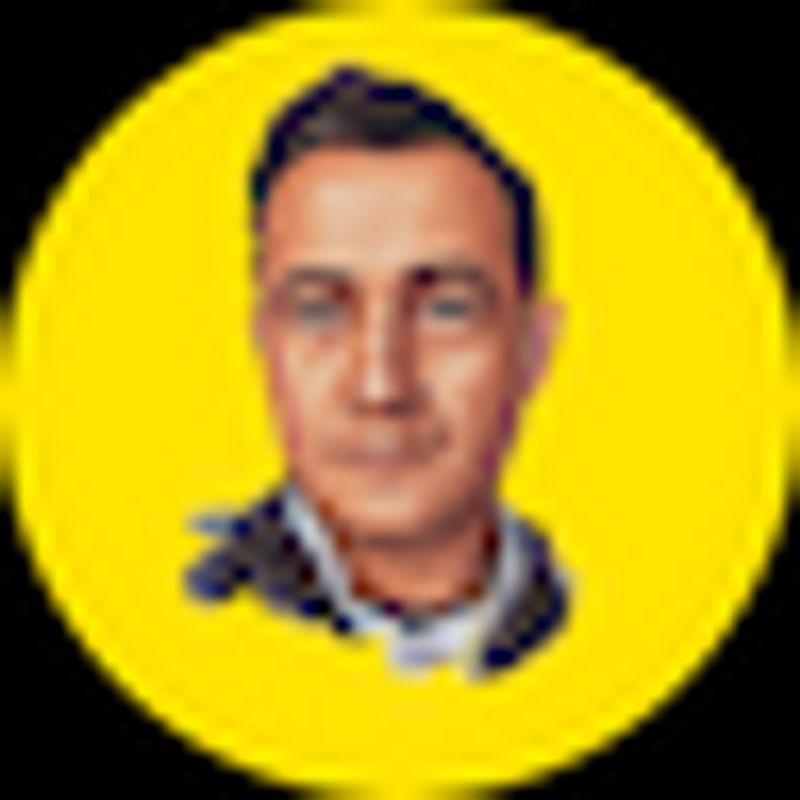
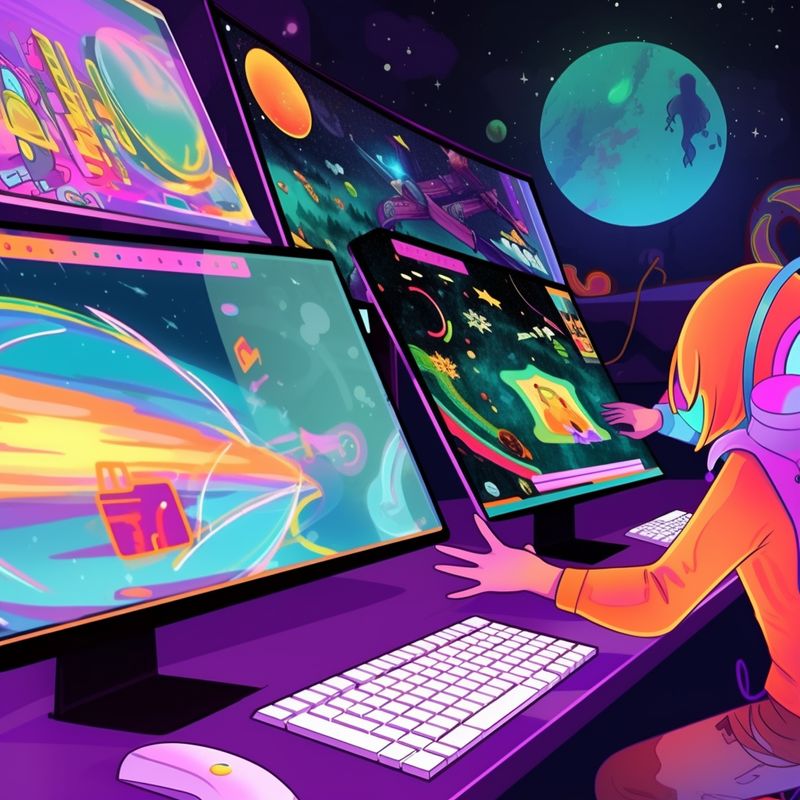
Create Root Layout
First, we need to create a root layout file (layout.svelte
) in our src/routes
directory. This file will contain the navigation bar and the PageTransition
component that will wrap our routes.
Svelte<script> import PageTransition from '../lib/components/PageTransition.svelte'; export let data; $: ({ pathname } = data); </script> <nav> <a href="/" class:active={pathname === '/'}>Home</a> <a href="/host/homes" class:active={pathname === '/host/homes'}>Airbnb your home</a> </nav> <PageTransition {pathname}> <slot /> </PageTransition> <style> nav { display: flex; justify-content: space-between; align-items: center; padding: 1rem; background-color: #fff; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } a { color: rgb(15, 134, 134); text-decoration: none; } a:hover { text-decoration: underline; } .active { color: rgb(9, 90, 90); font-weight: bold; } </style>
Export load method for root layout
We need to export a load
method in the corresponding +layout.ts
file in order to make available the pathname
binding available on the root page data
prop. This binding will change whenever a page transition takes place and thus triggers our page transition animation.
TypeScriptexport const load = async ({ url }) => { return { pathname: url.pathname }; };
Create PageTransition component
Finally we create the PageTransition
component in src/lib/components
.
Svelte<script lang="ts"> import { fade } from 'svelte/transition'; import { cubicInOut } from 'svelte/easing'; export let pathname: string; const duration = 150; </script> {#key pathname} <div in:fade={{ delay: duration, easing: cubicInOut }} out:fade={{ duration, easing: cubicInOut }} > <slot /> </div> {/key}
Add other page route
Create the dummy page that we will transition to from the home page in src/routes/host/homes
directory.
Svelte<div> <h1>Airbnb your home</h1> </div> <style> div { display: grid; place-items: center; height: 100vh; } h1 { font-size: 2rem; font-weight: 500; } </style>
Wrap Up
That’s it. Now we have a fancy page transition.
Check out the sample repo and the deployed site. Ask questions in the comment section!